Crate sea_orm
Expand description
§SeaORM
§SeaORM is a relational ORM to help you build web services in Rust with the familiarity of dynamic languages.
If you like what we do, consider starring, sharing and contributing!
Please help us with maintaining SeaORM by completing the SeaQL Community Survey 2024!
Join our Discord server to chat with other members of the SeaQL community!
§Getting Started
Integration examples:
- Actix v4 Example
- Axum Example
- GraphQL Example
- jsonrpsee Example
- Loco TODO Example / Loco REST Starter
- Poem Example
- Rocket Example / Rocket OpenAPI Example
- Salvo Example
- Tonic Example
- Seaography Example
§Features
-
Async
Relying on SQLx, SeaORM is a new library with async support from day 1.
-
Dynamic
Built upon SeaQuery, SeaORM allows you to build complex dynamic queries.
-
Service Oriented
Quickly build services that join, filter, sort and paginate data in REST, GraphQL and gRPC APIs.
-
Production Ready
SeaORM is feature-rich, well-tested and used in production by companies and startups.
§A quick taste of SeaORM
§Entity
use sea_orm::entity::prelude::*;
#[derive(Clone, Debug, PartialEq, DeriveEntityModel)]
#[sea_orm(table_name = "cake")]
pub struct Model {
#[sea_orm(primary_key)]
pub id: i32,
pub name: String,
}
#[derive(Copy, Clone, Debug, EnumIter, DeriveRelation)]
pub enum Relation {
#[sea_orm(has_many = "super::fruit::Entity")]
Fruit,
}
impl Related<super::fruit::Entity> for Entity {
fn to() -> RelationDef {
Relation::Fruit.def()
}
}
§Select
// find all models
let cakes: Vec<cake::Model> = Cake::find().all(db).await?;
// find and filter
let chocolate: Vec<cake::Model> = Cake::find()
.filter(cake::Column::Name.contains("chocolate"))
.all(db)
.await?;
// find one model
let cheese: Option<cake::Model> = Cake::find_by_id(1).one(db).await?;
let cheese: cake::Model = cheese.unwrap();
// find related models (lazy)
let fruits: Vec<fruit::Model> = cheese.find_related(Fruit).all(db).await?;
// find related models (eager)
let cake_with_fruits: Vec<(cake::Model, Vec<fruit::Model>)> =
Cake::find().find_with_related(Fruit).all(db).await?;
§Insert
let apple = fruit::ActiveModel {
name: Set("Apple".to_owned()),
..Default::default() // no need to set primary key
};
let pear = fruit::ActiveModel {
name: Set("Pear".to_owned()),
..Default::default()
};
// insert one
let pear = pear.insert(db).await?;
// insert many
Fruit::insert_many([apple, pear]).exec(db).await?;
§Update
use sea_orm::sea_query::{Expr, Value};
let pear: Option<fruit::Model> = Fruit::find_by_id(1).one(db).await?;
let mut pear: fruit::ActiveModel = pear.unwrap().into();
pear.name = Set("Sweet pear".to_owned());
// update one
let pear: fruit::Model = pear.update(db).await?;
// update many: UPDATE "fruit" SET "cake_id" = NULL WHERE "fruit"."name" LIKE '%Apple%'
Fruit::update_many()
.col_expr(fruit::Column::CakeId, Expr::value(Value::Int(None)))
.filter(fruit::Column::Name.contains("Apple"))
.exec(db)
.await?;
§Save
let banana = fruit::ActiveModel {
id: NotSet,
name: Set("Banana".to_owned()),
..Default::default()
};
// create, because primary key `id` is `NotSet`
let mut banana = banana.save(db).await?;
banana.name = Set("Banana Mongo".to_owned());
// update, because primary key `id` is `Set`
let banana = banana.save(db).await?;
§Delete
// delete one
let orange: Option<fruit::Model> = Fruit::find_by_id(1).one(db).await?;
let orange: fruit::Model = orange.unwrap();
fruit::Entity::delete(orange.into_active_model())
.exec(db)
.await?;
// or simply
let orange: Option<fruit::Model> = Fruit::find_by_id(1).one(db).await?;
let orange: fruit::Model = orange.unwrap();
orange.delete(db).await?;
// delete many: DELETE FROM "fruit" WHERE "fruit"."name" LIKE 'Orange'
fruit::Entity::delete_many()
.filter(fruit::Column::Name.contains("Orange"))
.exec(db)
.await?;
§🧭 Seaography: instant GraphQL API
Seaography is a GraphQL framework built on top of SeaORM. Seaography allows you to build GraphQL resolvers quickly. With just a few commands, you can launch a GraphQL server from SeaORM entities!
Look at the Seaography Example to learn more.
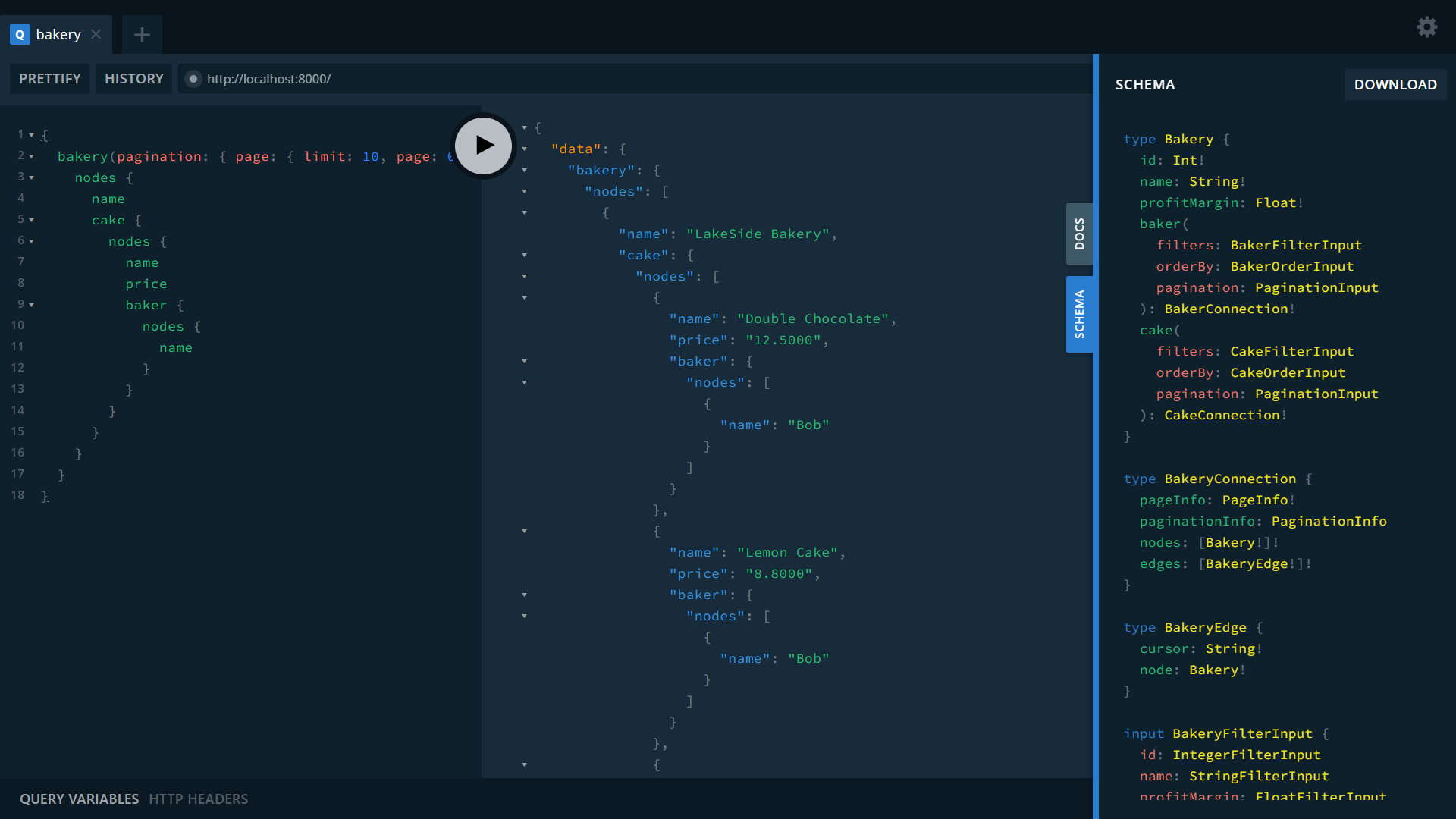
§🖥️ SeaORM Pro: Effortless Admin Panel
SeaORM Pro is an admin panel solution allowing you to quickly and easily launch an admin panel for your application - frontend development skills not required, but certainly nice to have!
Features:
- Full CRUD
- Built on React + GraphQL
- Built-in GraphQL resolver
- Customize the UI with simple TOML
Learn More
§Releases
SeaORM 1.0 is a stable release. The 1.x version will be updated until at least October 2025, and we’ll decide whether to release a 2.0 version or extend the 1.x life cycle.
It doesn’t mean that SeaORM is ‘done’, we’ve designed an architecture to allow us to deliver new features without major breaking changes. In fact, more features are coming!
§Who’s using SeaORM?
Here is a short list of awesome open source software built with SeaORM. Full list here. Feel free to submit yours!
Project | GitHub | Tagline |
---|---|---|
Zed | A high-performance, multiplayer code editor | |
OpenObserve | Open-source observability platform | |
RisingWave | Stream processing and management platform | |
LLDAP | A light LDAP server for user management | |
Warpgate | Smart SSH bastion that works with any SSH client | |
Svix | The enterprise ready webhooks service | |
Ryot | The only self hosted tracker you will ever need | |
Lapdev | Self-hosted remote development enviroment | |
System Initiative | DevOps Automation Platform | |
OctoBase | A light-weight, scalable, offline collaborative data backend |
§License
Licensed under either of
- Apache License, Version 2.0 (LICENSE-APACHE or http://www.apache.org/licenses/LICENSE-2.0)
- MIT license (LICENSE-MIT or http://opensource.org/licenses/MIT)
at your option.
§Contribution
Unless you explicitly state otherwise, any contribution intentionally submitted for inclusion in the work by you, as defined in the Apache-2.0 license, shall be dual licensed as above, without any additional terms or conditions.
We invite you to participate, contribute and together help build Rust’s future.
A big shout out to our contributors!
§Sponsorship
SeaQL.org is an independent open-source organization run by passionate developers. If you enjoy using our libraries, please star and share our repositories. If you feel generous, a small donation via GitHub Sponsor will be greatly appreciated, and goes a long way towards sustaining the organization.
§Silver Sponsors
|
|
We’re immensely grateful to our sponsors: Digital Ocean, for sponsoring our servers. And JetBrains, for sponsoring our IDE.
§Mascot
A friend of Ferris, Terres the hermit crab is the official mascot of SeaORM. His hobby is collecting shells.
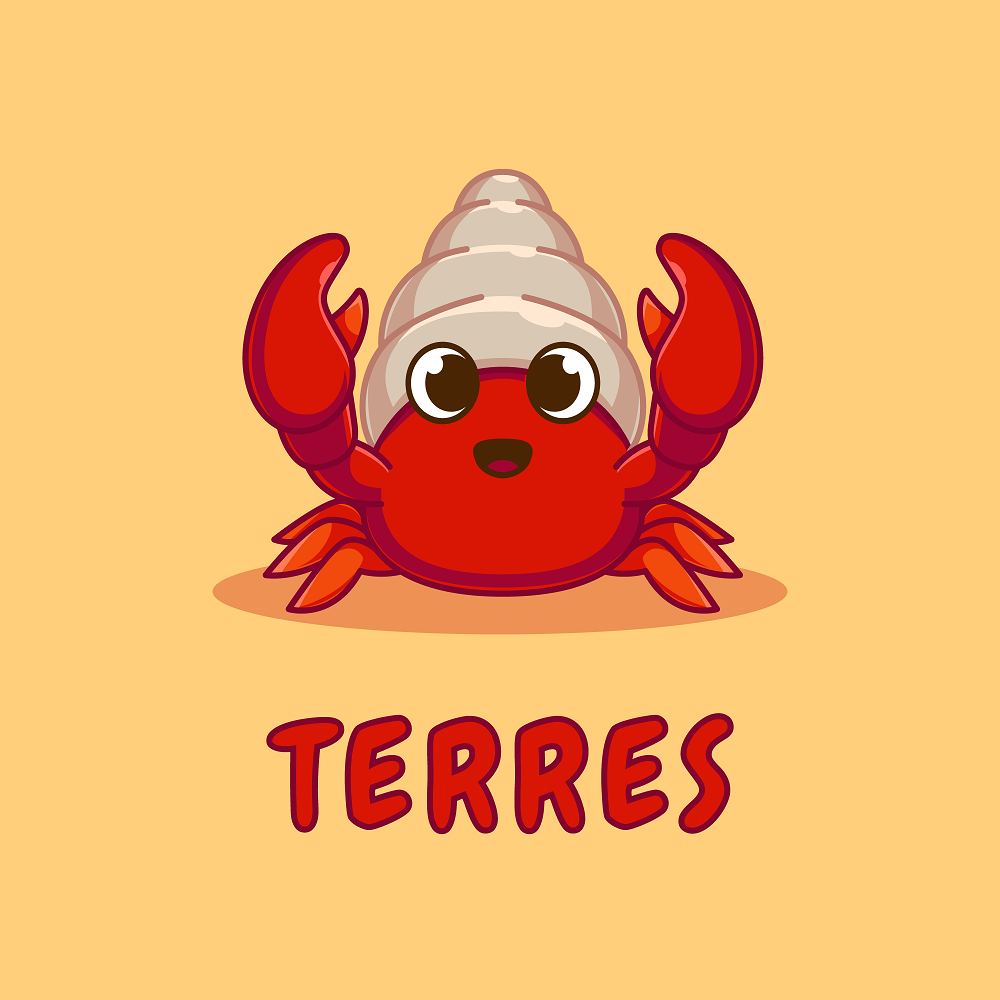
Modules§
- entity
- Module for the Entity type and operations
- error
- Error types for all database operations
- metric
- Holds types and methods to perform metric collection
- query
- Holds types and methods to perform queries
- schema
- Holds types that defines the schemas of an Entity
- sea_
query - sqlx
- The async SQL toolkit for Rust, built with ❤️ by the LaunchBadge team.
- strum
- Strum
Macros§
- debug_
print - Uses the
log
crate to perform logging. This must be enabled using the feature flagdebug-print
. - debug_
query - Helper to get a raw SQL string from an object that impl
QueryTrait
. - debug_
query_ stmt - Helper to get a
Statement
from an object that implQueryTrait
.
Structs§
- Column
Def - Defines a Column for an Entity
- Column
From StrErr - Error during
impl FromStr for Entity::Column
- Condition
- Represents the value of an
Condition::any
orCondition::all
: a set of disjunctive or conjunctive conditions. - Connect
Options - Defines the configuration options of a database
- Cursor
- Cursor pagination
- Database
- Defines a database
- Database
Transaction - Defines a database transaction, whether it is an open transaction and the type of backend to use
- Debug
Query - This structure provides debug capabilities
- Delete
- Defines the structure for a delete operation
- Delete
Many - Perform a delete operation on multiple models
- Delete
One - Perform a delete operation on a model
- Delete
Result - The result of a DELETE operation
- Deleter
- Handles DELETE operations in a ActiveModel using DeleteStatement
- Exec
Result - Defines the result of executing an operation
- Insert
- Performs INSERT operations on a ActiveModel
- Insert
Result - The result of an INSERT operation on an ActiveModel
- Inserter
- Defines a structure to perform INSERT operations in an ActiveModel
- Items
AndPages Number - Define a structure containing the numbers of items and pages of a Paginator
- Paginator
- Defined a structure to handle pagination of a result from a query operation on a Model
- Query
Result - Defines the result of a query operation on a Model
- Query
Stream - The self-referencing struct.
- Relation
Builder - Defines a helper to build a relation
- Relation
Def - Defines a relationship
- Schema
- This is a helper struct to convert
EntityTrait
into differentsea_query
statements. - Select
- Defines a structure to perform select operations
- SelectA
- Implements the traits Iden and IdenStatic for a type
- SelectB
- Implements the traits Iden and IdenStatic for a type
- SelectC
- Implements the traits Iden and IdenStatic for a type
- Select
Getable Tuple - Get tuple from query result based on column index
- Select
Getable Value - Get tuple from query result based on a list of column identifiers
- Select
Model - Helper class to handle query result for 1 Model
- Select
Three - Defines a structure to perform a SELECT operation on two Models
- Select
Three Model - Helper class to handle query result for 3 Models
- Select
Two - Defines a structure to perform a SELECT operation on two Models
- Select
TwoMany - Defines a structure to perform a SELECT operation on many Models
- Select
TwoModel - Helper class to handle query result for 2 Models
- Selector
- Defines a type to do
SELECT
operations through a SelectStatement on a Model - Selector
Raw - Performs a raw
SELECT
operation on a model - Sqlx
Sqlite Connector - Defines the sqlx::sqlite connector
- Sqlx
Sqlite Error - Sqlx
Sqlite Pool Connection - Defines a sqlx SQLite pool
- Statement
- Defines an SQL statement
- Transaction
Stream - The self-referencing struct.
- TryInsert
- Performs INSERT operations on a ActiveModel, will do nothing if input is empty.
- Update
- Defines a structure to perform UPDATE query operations on a ActiveModel
- Update
Many - Defines an UPDATE operation on multiple ActiveModels
- Update
One - Defines an UPDATE operation on one ActiveModel
- Update
Result - The result of an update operation on an ActiveModel
- Updater
- Defines an update operation
- Values
Enums§
- Access
Mode - Access mode
- Active
Value - Defines a stateful value used in ActiveModel.
- Column
Type - All column types
- Conn
Acquire Err - Connection Acquire error
- Database
Backend - The type of database backend for real world databases. This is enabled by feature flags as specified in the crate documentation
- Database
Connection - Handle a database connection depending on the backend enabled by the feature
flags. This creates a database pool. This will be
Clone
unless the feature flagmock
is enabled. - DbErr
- An error from unsuccessful database operations
- Identity
- List of column identifier
- Isolation
Level - Isolation level
- Join
Type - Join types
- Json
Value - Represents any valid JSON value.
- Order
- Ordering options
- Relation
Type - Defines the type of relationship
- Runtime
Err - Runtime error
- SqlErr
- An error from unsuccessful SQL query
- Sqlx
Error - Represents all the ways a method can fail within SQLx.
- Transaction
Error - Defines errors for handling transaction failures
- TryGet
Error - An error from trying to get a row from a Model
- TryInsert
Result - The types of results for an INSERT operation
- Value
- Value variants
Traits§
- Active
Enum - A Rust representation of enum defined in database.
- Active
Enum Value - The Rust Value backing ActiveEnums
- Active
Model Behavior - A Trait for overriding the ActiveModel behavior
- Active
Model Trait - A Trait for ActiveModel to perform Create, Update or Delete operation. The type must also implement the EntityTrait. See module level docs crate::entity for a full example
- ColIdx
- Column Index, used by
TryGetable
. Implemented for&str
andusize
- Column
Trait - API for working with a
Column
. Mostly a wrapper of the identically named methods insea_query::Expr
- Column
Type Trait - SeaORM’s utility methods that act on ColumnType
- Conditional
Statement - Connection
Trait - The generic API for a database connection that can perform query or execute statements. It abstracts database connection and transaction
- Cursor
Trait - A trait for any type that can be turn into a cursor
- Entity
Name - A Trait for mapping an Entity to a database table
- Entity
OrSelect - Entity, or a Select
; to be used as parameters in LoaderTrait
- Entity
Trait - An abstract base class for defining Entities.
- From
Query Result - A Trait for implementing a QueryResult
- Iden
- Identifier
- Iden
Static - Ensure the identifier for an Entity can be converted to a static str
- Identity
Of - Check the Identity of an Entity
- Into
Active Model - A Trait for any type that can be converted into an ActiveModel
- Into
Active Value - Any type that can be converted into an ActiveValue
- Into
Identity - Performs a conversion into an Identity
- Into
Simple Expr - Performs a conversion to SimpleExpr
- Iterable
- This trait designates that an
Enum
can be iterated over. It can be auto generated using theEnumIter
derive macro. - Linked
- A Trait for links between Entities
- Loader
Trait - This trait implements the Data Loader API
- Model
Trait - The interface for Model, implemented by data structs
- Ordered
Statement - Paginator
Trait - A Trait for any type that can paginate results
- Partial
Model Trait - A trait for a part of Model
- Primary
KeyArity - How many columns this Primary Key comprises
- Primary
KeyTo Column - How to map a Primary Key to a column
- Primary
KeyTrait - A Trait for to be used to define a Primary Key.
- Query
Filter - Perform a FILTER opertation on a statement
- Query
Order - Performs ORDER BY operations
- Query
Select - Abstract API for performing queries
- Query
Trait - A Trait for any type performing queries on a Model or ActiveModel
- Related
- Checks if Entities are related
- Relation
Trait - Defines the relations of an Entity
- Select
Columns - Select specific column for partial model queries
- Selector
Trait - A Trait for any type that can perform SELECT queries
- Statement
Builder - Any type that can build a Statement
- Stream
Trait - Stream query results
- Transaction
Trait - Spawn database transaction
- TryFrom
U64 - Try to convert a type to a u64
- TryGetable
- An interface to get a value from the query result
- TryGetable
Array - An interface to get an array of values from the query result.
A type can only implement
ActiveEnum
orTryGetableFromJson
, but not both. A blanket impl is provided forTryGetableFromJson
, while the impl forActiveEnum
is provided by theDeriveActiveEnum
macro. So as an end user you won’t normally touch this trait. - TryGetable
From Json - An interface to get a JSON from the query result
- TryGetable
Many - An interface to get a tuple value from the query result
- TryInto
Model - A Trait for any type that can be converted into an Model
Functions§
- Unset
Deprecated - Defines an not set operation on an ActiveValue
Type Aliases§
- DbBackend
- The same as DatabaseBackend just shorter :)
- DbConn
- The same as a DatabaseConnection
- DynIden
- Foreign
KeyAction - Action to perform on a foreign key whenever there are changes to an ActiveModel
- LinkDef
- Same as RelationDef
- PinBox
Stream - Pin a Model so that stream operations can be performed on the model
Derive Macros§
- Derive
Active Enum - A derive macro to implement
sea_orm::ActiveEnum
trait for enums. - Derive
Active Model - The DeriveActiveModel derive macro will implement ActiveModelTrait for ActiveModel which provides setters and getters for all active values in the active model.
- Derive
Active Model Behavior - Models that a user can override
- Derive
Column - The DeriveColumn derive macro will implement [ColumnTrait] for Columns. It defines the identifier of each column by implementing Iden and IdenStatic. The EnumIter is also derived, allowing iteration over all enum variants.
- Derive
Custom Column - Derive a column if column names are not in snake-case
- Derive
Display - Derive
Entity - Create an Entity
- Derive
Entity Model - This derive macro is the ‘almighty’ macro which automatically generates Entity, Column, and PrimaryKey from a given Model.
- Derive
Iden - The DeriveIden derive macro will implement
sea_orm::sea_query::Iden
for simplify Iden implementation. - Derive
Into Active Model - Derive into an active model
- Derive
Migration Name - The DeriveMigrationName derive macro will implement
sea_orm_migration::MigrationName
for a migration. - Derive
Model - The DeriveModel derive macro will implement ModelTrait for Model, which provides setters and getters for all attributes in the mod It also implements FromQueryResult to convert a query result into the corresponding Model.
- Derive
Partial Model - The DerivePartialModel derive macro will implement [
sea_orm::PartialModelTrait
] for simplify partial model queries. - Derive
Primary Key - The DerivePrimaryKey derive macro will implement [PrimaryKeyToColumn] for PrimaryKey which defines tedious mappings between primary keys and columns. The EnumIter is also derived, allowing iteration over all enum variants.
- Derive
Related Entity - The DeriveRelatedEntity derive macro will implement seaography::RelationBuilder for RelatedEntity enumeration.
- Derive
Relation - The DeriveRelation derive macro will implement RelationTrait for Relation.
- Derive
Value Type - Implements traits for types that wrap a database value type.
- Enum
Iter - Creates a new type that iterates of the variants of an enum.
- From
Json Query Result - From
Query Result - Convert a query result into the corresponding Model.
- Iden